Example of Set and operations as follows:
//Program tested on Microsoft Visual Studio 2008 - Zahid Ghadialy
#include<iostream>
#include <set>
using namespace std;
int main()
{
int someInts[] = {3, 6, 9, 12, 15, 18};
int someIntsSize = sizeof(someInts) / sizeof(int);
set<int> someSet (someInts, someInts + someIntsSize);
cout<<"\n1. someSet : ";
copy(someSet.begin(), someSet.end(), ostream_iterator<int>(cout, " "));
cout<<endl;
//Insert some more elements
someSet.insert(0);
someSet.insert(10);
someSet.insert(20);
cout<<"\n2. someSet : ";
copy(someSet.begin(), someSet.end(), ostream_iterator<int>(cout, " "));
cout<<endl;
//Upper Bound means the next greater number
set<int>::iterator it = someSet.upper_bound(5);
cout << "\nupper_bound(5) = " << *it << endl;
it = someSet.upper_bound(10);
cout << "\nupper_bound(10) = " << *it << endl;
//Lower bound means either equal or greater number
it = someSet.lower_bound(5);
cout << "\nlower_bound(5) = " << *it << endl;
it = someSet.lower_bound(10);
cout << "\nlower_bound(10) = " << *it << endl;
//Equal Range returns a pair
pair<set<int>::iterator,set<int>::iterator> retIt;
retIt = someSet.equal_range(5);
cout<<"\nequal_range(5) - lower bound = " << *retIt.first <<
" upper bound = " << *retIt.second << endl;
retIt = someSet.equal_range(10);
cout<<"\nequal_range(10) - lower bound = " << *retIt.first <<
" upper bound = " << *retIt.second << endl;
cout<<endl;
return 0;
}
The output is as follows:
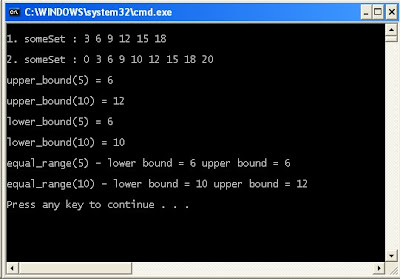
good one.
ReplyDeleteQuick question: Have a employee database which is to be searched by emp id or ssn to retrieve their employment detais/ Which c++ data strucuter can be used for this problem. Any pointers would help.
ReplyDeleteGenerally you would define a class with all the employee details, etc and then use a Map to map an instance of this class against the emp id. In fact you can create multiple maps where you can store the same instance of the employee class and be able to sort using emp id in one map and ssn on other map, etc. Assuming that emp id and ssn are usnique. If not then you will have to use something else or modify the code accordingly.
ReplyDelete