For some reason many C++ programmers believe there is an isNumeric() function available as part of the language. In fact I was searching for the header file to include to get a piece of code to work.
Its not difficult to create a function that will be able to check if the string is numeric or not. Here is the code.
//Program tested on Microsoft Visual Studio 2008 - Zahid Ghadialy
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
bool isNumeric1(string stringToCheck)
{
bool numeric = false;
if(stringToCheck.find_first_not_of("0123456789.") == string::npos)
numeric = true;
return numeric;
}
bool isNumeric2(string stringToCheck)
{
bool numeric = true;
unsigned i = 0;
while(numeric && i < stringToCheck.length())
{
switch (stringToCheck[i])
{
case '0': case '1': case '2': case '3': case '4': case '5':
case '6': case '7': case '8': case '9': case '.':
//do nothing
break;
default:
numeric = false;
}
i++;
}
return numeric;
}
bool isNumeric3(string stringToCheck)
{
stringstream streamVal(stringToCheck);
double tempVal;
streamVal >> tempVal; //If numeric then everything transferred to tempVal
if(streamVal.get() != EOF)
return false;
else
return true;
}
int main()
{
string str1("123"), str2("134.567"), str3("12AB");
cout << "Approach 1" << endl;
cout << str1 << (isNumeric1(str1) ? " is Numeric" : " is Not Numeric") << endl;
cout << str2 << (isNumeric1(str2) ? " is Numeric" : " is Not Numeric") << endl;
cout << str3 << (isNumeric1(str3) ? " is Numeric" : " is Not Numeric") << endl;
cout << "\nApproach 2" << endl;
cout << str1 << (isNumeric2(str1) ? " is Numeric" : " is Not Numeric") << endl;
cout << str2 << (isNumeric2(str2) ? " is Numeric" : " is Not Numeric") << endl;
cout << str3 << (isNumeric2(str3) ? " is Numeric" : " is Not Numeric") << endl;
cout << "\nApproach 3" << endl;
cout << str1 << (isNumeric3(str1) ? " is Numeric" : " is Not Numeric") << endl;
cout << str2 << (isNumeric3(str2) ? " is Numeric" : " is Not Numeric") << endl;
cout << str3 << (isNumeric3(str3) ? " is Numeric" : " is Not Numeric") << endl;
return 0;
}
Output is as follows:
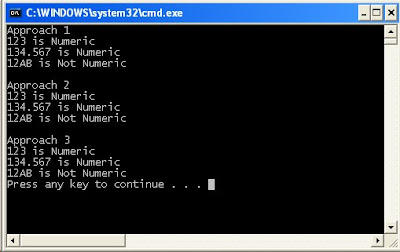
There are few problems in the program above:
1. It does not cater for negative numbers
2. It will return wrong result when you have incorrect string like 12.34.56.78
I have intentionally left it as an exercise.
Other approaches are possible as well. Why not try and think of an approach yourself.
very nice blog with very useful examples. great work keep it up!
ReplyDeleteActually, there is something that supposedly does this..
ReplyDeleteThough I haven't tested it...
Header File: ctype.h
Function Name: isdigit()
This function accepts an ASCII value, and returns whether or not it is a digit (0 to 9) when converted to its equivalent ASCII character. It returns a zero if it is not a digit, and non-zero if it is.