//Program tested on Microsoft Visual Studio 2008 - Zahid Ghadialy
#include<iostream>
using namespace std;
class Parent
{
public:
virtual void func_a(void);
};
class Child : public Parent
{
public:
void func_a(void);
};
void func_1(Parent p);
void func_2(Parent* p);
void func_3(Parent& p);
int main()
{
//Using the Parent class
Parent p;
cout<<"\nParent Class"<<endl;
func_1(p);
func_2(&p);
func_3(p);
//Using the Child class
Child c;
cout<<"\n\nChild Class"<<endl;
func_1(c);
func_2(&c);
func_3(c);
//Using the Parent class that is initialised to class
Parent p2 = c; //Note that in p2, additional info about c would be lost
cout<<"\n\nParent p2 Class"<<endl;
func_1(p2);
func_2(&p2);
func_3(p2);
//Not possible to initialise directly from base to derived class.
//Child c2 = p2; - ERROR: cannot convert from 'Parent' to 'Child'
//The way round it would be to have a constructor or overload operator =
return 0;
}
void Parent::func_a()
{
cout<<"Parent::func_a()"<<endl;
}
void Child::func_a(void)
{
cout<<"Child::func_a()"<<endl;
}
void func_1(Parent p)
{
cout<<"func_1 -> ";
p.func_a();
}
void func_2(Parent* p)
{
cout<<"func_2 -> ";
p->func_a();
}
void func_3(Parent& p)
{
cout<<"func_3 -> ";
p.func_a();
}
The output is as follows:
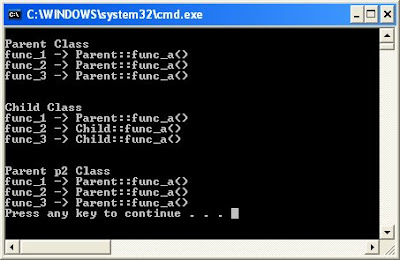
No comments:
Post a Comment