//Program tested on Microsoft Visual Studio 2008 - Zahid Ghadialy
//Program to show how to use variable number of arguments
#include<iostream>
#include <stdarg.h> //needed for va_ macros
using namespace std;
//List Should be terminated by -1 in our case
int Add(int a,...)
{
int total = a;
int l_ParamVal=0;
//Declare a va_list macro and initialize it with va_start
va_list l_Arg;
va_start(l_Arg,a);
while((l_ParamVal = va_arg(l_Arg,int)) != -1)
{
total+= l_ParamVal;
}
va_end(l_Arg);
return total;
}
int main()
{
cout<<"Result of Add(2) = "<<Add(2, -1)<<endl;
cout<<"Result of Add(2, 3) = "<<Add(2, 3, -1)<<endl;
cout<<"Result of Add(2, 3, 31) = "<<Add(2, 3, 31, -1)<<endl;
cout<<"Result of Add(2, 3, 31, 77) = "<<Add(2, 3, 31, 77, -1)<<endl;
cout<<endl;
return 0;
}
The output is as follows:
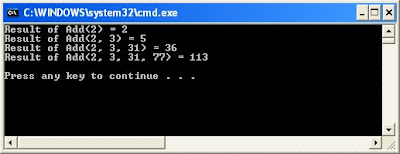
No comments:
Post a Comment