//Program tested on Microsoft Visual Studio 2008 - Zahid Ghadialy
//This example shows how some people abuse throw and catch
#include<iostream>
using namespace std;
//
//The following interface is defined and being used by many classes
void function1();
//Now some programmer wants to change this to
//int function1();
//But this is not possible since overloading does not work for return types
//
//The same problem could instead be solved by other approach as follows:
void function1(int &abc);
int function1(int dummy1, int dummy2); /*Note 2 dummy to avoid ambiguity with
the first overload. If the above overloaded func was not
there then only 1 dummy is sufficient
*/
int main()
{
try
{
function1();
}
catch(int xyz)
{
cout<<"\n\n";
cout<<"The function1() returned "<<xyz<<endl;
cout<<"\n\n";
}
int first;
function1(first);
cout<<"The 1st overloaded function1() returned "<<first<<endl;
cout<<"\n\n";
cout<<"The 2nd overloaded function1() returned "<<function1(3,4)<<endl;
cout<<"\n\n";
return 0;
}
void function1()
{
//function logic
throw 100;
}
//Overloaded function1()
void function1(int &abc)
{
abc = 120;
}
//Overloaded function1()
int function1(int dummy1, int dummy2)
{
return 140;
}
The output is as follows:
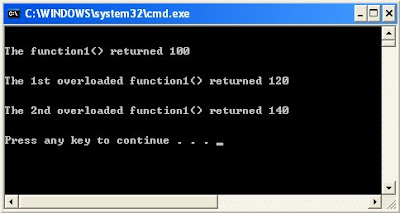
No comments:
Post a Comment