//Program tested on Microsoft Visual Studio 2008 - Zahid Ghadialy
//This program shows how to create vectors, check size and capacity,
//change the value of the elements and finally clear the vector
#include <iostream>
#include <vector>
using namespace std;
int main()
{
//Create a vector called vectorOne
vector<int> vectorOne; //Note size is 0
//Resize to 3 with default value 7
vectorOne.resize(3,7);
//Print the output to see the resize
cout<<"Test 1"<<endl;
for(unsigned i=0;i<vectorOne.size();i++)
{
cout<<"Element: "<<i<<" Value: "<<vectorOne.at(i)<<endl;
}
//Resize further to 7 and new values default to 4
vectorOne.resize(7,4);
cout<<"\nTest 2"<<endl;
for(unsigned i=0; i<vectorOne.size(); i++)
{
cout<<"Element: "<<i<<" Value: "<<vectorOne.at(i)<<endl;
}
//Check what the size and capacity of the vector is
cout<<endl;
cout<<"Size of vectorOne is: "<<vectorOne.size()<<endl;
cout<<"Capacity of vectorOne is: "<<vectorOne.capacity()<<endl;
//Lets modify the values of these vectors
for(unsigned i=0,j=22;i<vectorOne.size();i++,j+=i)
{
vectorOne.at(i)=j;
}
cout<<"\nTest 3"<<endl;
for(unsigned i=0; i<vectorOne.size(); i++)
{
cout<<"Element: "<<i<<" Value: "<<vectorOne.at(i)<<endl;
}
//Use reserve to reallocate vectorOne with enough storage for 10 elements
vectorOne.reserve(10);
cout<<"\nSize of vectorOne is: "<<vectorOne.size()<<endl;
cout<<"Capacity of vectorOne is: "<<vectorOne.capacity()<<endl;
//Try resizing to 15 and dont add any default values for the new elements
vectorOne.resize(15);
cout<<"\nSize of vectorOne is: "<<vectorOne.size()<<endl;
cout<<"Capacity of vectorOne is: "<<vectorOne.capacity()<<endl;
//Remove all elements of the vector
vectorOne.clear();
cout<<"\nVector Cleared"<<endl;
cout<<"Size of vectorOne is: "<<vectorOne.size()<<endl;
cout<<"Capacity of vectorOne is: "<<vectorOne.capacity()<<endl;
cout<<endl;
return 0;
}
The output of the program is as follows:
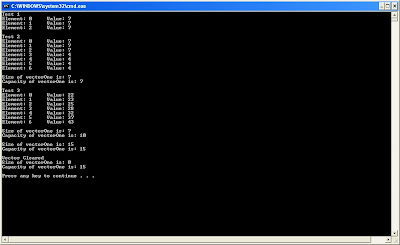
No comments:
Post a Comment