//Program tested on Microsoft Visual Studio 2008 - Zahid Ghadialy
#include <iostream>
#include <vector>
#include <string>
#include <algorithm> //needed for std::search
using namespace std;
bool isOdd(int i)
{
return ((i%2)==1);
}
int main()
{
vector<int> v1;
v1.push_back(88);
v1.push_back(99);
v1.push_back(22);
v1.push_back(33);
v1.push_back(44);
v1.push_back(55);
v1.push_back(66);
v1.push_back(77);
//Demonstrating Search - Matching Case
vector<int> v2;
v2.push_back(22);
v2.push_back(33);
v2.push_back(44);
vector<int>::const_iterator it = search(v1.begin(), v1.end(), v2.begin(), v2.end());
if(it != v1.end())
{
cout<<"Found: "<<*it<<endl;
cout<<"Position = "<<it - v1.begin() + 1<<endl;
}
else
{
cout<<"Not Found"<<endl;
cout<<"Position = -1"<<endl;
}
//Demonstrating Search - Non-Matching Case
vector<int> v3;
v3.push_back(22);
v3.push_back(33);
v3.push_back(444); //non matching
it = search(v1.begin(), v1.end(), v3.begin(), v3.end());
if(it != v1.end())
{
cout<<"Found: "<<*it<<endl;
cout<<"Position = "<<it - v1.begin() + 1<<endl;
}
else
{
cout<<"Not Found"<<endl;
cout<<"Position = -1"<<endl;
}
//Use the search to find strings
string s1="Hello, this is Zahid";
//Matching case
string s2="Zahid";
string::const_iterator it2 = search(s1.begin(), s1.end(), s2.begin(), s2.end());
if(it2 != s1.end())
{
cout<<"Found: "<<*it2<<endl;
cout<<"Position = "<<it2 - s1.begin() + 1<<endl;
}
else
{
cout<<"Not Found"<<endl;
cout<<"Position = -1"<<endl;
}
//Non-Matching case
string s3="None";
it2 = search(s1.begin(), s1.end(), s3.begin(), s3.end());
if(it2 != s1.end())
{
cout<<"Found: "<<*it2<<endl;
cout<<"Position = "<<it2 - s1.begin() + 1<<endl;
}
else
{
cout<<"Not Found"<<endl;
cout<<"Position = -1"<<endl;
}
return 0;
}
The output is as follows:
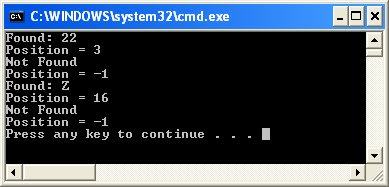